Watching - An Intangible Medium Interactive Art Installation The final Interactive Art Installation is complete.…
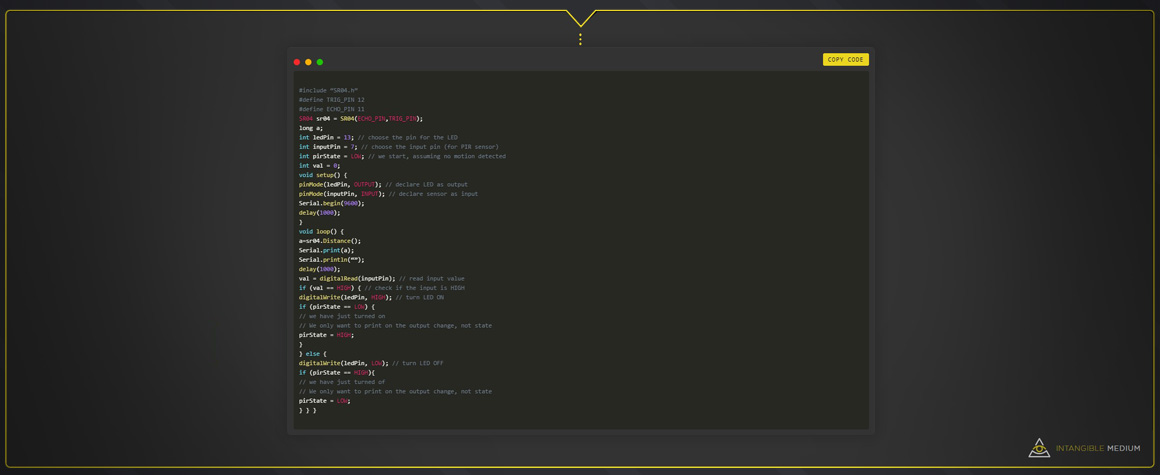
Watching | p5.js Sketch & Arduino Sensor Code
Here you’ll find the code base for the “Watching” interactive art installation. The code could be more elegant, but it will definitely be refined overtime.
Arduino interactive art installation sensor control IDE sketch code:
#include “SR04.h”
#define TRIG_PIN 12
#define ECHO_PIN 11
SR04 sr04 = SR04(ECHO_PIN,TRIG_PIN);
long a;
int ledPin = 13; // choose the pin for the LED
int inputPin = 7; // choose the input pin (for PIR sensor)
int pirState = LOW; // we start, assuming no motion detected
int val = 0;
void setup() {
pinMode(ledPin, OUTPUT); // declare LED as output
pinMode(inputPin, INPUT); // declare sensor as input
Serial.begin(9600);
delay(1000);
}
void loop() {
a=sr04.Distance();
Serial.print(a);
Serial.println(“”);
delay(1000);
val = digitalRead(inputPin); // read input value
if (val == HIGH) { // check if the input is HIGH
digitalWrite(ledPin, HIGH); // turn LED ON
if (pirState == LOW) {
// we have just turned on
// We only want to print on the output change, not state
pirState = HIGH;
}
} else {
digitalWrite(ledPin, LOW); // turn LED OFF
if (pirState == HIGH){
// we have just turned of
// We only want to print on the output change, not state
pirState = LOW;
} } }
p5.js interactive art installation sketch code:
let setSize; // Size
let setColor; // Color
let setSpeed; // Speed
let s = 0; // Speed equal to zero / zero equals noAnimated / >.01 equals isAnimated
let img; // Image
let capture; // Let Web Camera Capture
let font;
var x = 1;
var y = 1;
// Serial communication code forked from original code by Scott Fitzgerald youtube/github
// https://www.youtube.com/playlist?list=PLnkvii1uWBvEprzkCJZbSAWsiqncWodoQ
// https://gist.github.com/shfitz/7fd206b7db4e0e6416a443d61c8c988e
let serial; // variable for the serial object
let latestData = “waiting for data”; // variable to hold the data
function preload()
{img = loadImage(‘https://openprocessing-usercontent.s3.amazonaws.com/files/user230222/visual948744/ha96c880d1cccf73792bb9b42c8ef207f/im_logo.png’);
font = loadFont(‘gotham-bold.ttf’);
}
function setup() {
createCanvas(windowWidth, windowHeight);
capture = createCapture(VIDEO);
capture.size(720, 480);
textFont(font); // Load custom font
// Serial communication code —————————————————————————–
serial = new p5.SerialPort();
// get a list of all connected serial devices
serial.list();
// serial port to use – you’ll need to change this
serial.open(‘COM4’);
// callback for when the sketchs connects to the server
serial.on(‘connected’, serverConnected);
// callback to print the list of serial devices
serial.on(‘list’, gotList);
// what to do when we get serial data
serial.on(‘data’, gotData);
// what to do when there’s an error
serial.on(‘error’, gotError);
// when to do when the serial port opens
serial.on(‘open’, gotOpen);
// what to do when the port closes
serial.on(‘close’, gotClose);
function serverConnected() {
print(“Connected to Server”);
}
// list the ports
function gotList(thelist) {
print(“List of Serial Ports:”);
for (let i = 0; i < thelist.length; i++) {
print(i + ” ” + thelist[i]);
}
}
function gotOpen() {
print(“Serial Port is Open”);
}
function gotClose() {
print(“Serial Port is Closed”);
latestData = “Serial Port is Closed”;
}
function gotError(theerror) {
print(theerror);
}
// when data is received in the serial buffer
function gotData() {
let currentString = serial.readLine(); // store the data in a variable
trim(currentString); // get rid of whitespace
if (!currentString) return; // if there’s nothing in there, ignore it
console.log(currentString); // print it out
latestData = currentString; // save it to the global variable
}
// END // Serial communication code ———————————————————————–
noStroke();
image(img, width/2, height/2); //Set image/eye logo
}
function draw() {
background(0,33);
push();
tint(255, 7);
image(capture, width/2, height/2, windowWidth, windowHeight);
pop();
let sizeChanger = map(latestData, 0, 250, 16, 32); // Map size to canvas distance
setSize = sizeChanger; // Set Site
let speedChanger = map(233, 0, 250, 0.05, 0.02); // Map speed to canvas distance
setSpeed = speedChanger; // Set Speed
setColor = (“#ead621”); // Set Color
// Set color changer ————————————————————————————
fill(setColor);
// X and Y square particle grid ————————————————————————-
for (let x = 0; x <= windowWidth; x = x + 50) {
for (let y = 0; y <= windowHeight; y = y + 50) {
// Particle animated rotation ————————————————————————–
const xAngle = (0, 0, width, -PI * PI, PI * PI);
const yAngle = (0, 0, height, -PI * PI, PI * PI);
const angle = xAngle * (x / width) + yAngle * (y / height);
const setX = x + 33 * sin(PI * s + angle);
const setY = y + 33 * sin(PI * s + angle);
// Draw particle
ellipse (setX, setY, setSize);}}
// Set particle speed
s = s + setSpeed;
// UI background rectangle
fill(77, 77, 77, 177);
rectMode (CENTER);
ellipse (width/2,height/2,233,233);
let watching = map(latestData, 0, 80, 255, 0); // Map text fade to zero
fill(255,255,255,watching);
textSize(60);
textAlign (CENTER);
text(“WATCHING YOU”, width / 2, height / 2+200); // print the data to the sketch
// Insert image/eye logo ——————————————————————————–
imageMode (CENTER);
image(img, width/2, height/2-6);
// Draw logo eye dot ————————————————————————————
fill(setColor);
ellipse (width/2, height/2, 12);
// Draw distance value text —————————————————————————–
push();
fill(“#ead621”);
textSize(20);
textAlign (CENTER);
text(latestData, width / 2, height / 2+90); // print the data to the sketch
pop();
// Draw ellipse mask ————————————————————————————
push(); // Circle Mask
let c = map(“#ead621”, 0, 255, 255, 13); // Map color change with distance – Now hard set to #ead621
let fader = map(latestData, 0, 30, 255, 0); // Map color change with distance
fill(c, 30, 73, fader);
let d = map(latestData, 0, 300, 0, 230); // Map distance to canvas to ellipse size
ellipse(width / 2, height / 2, d, d);
pop();
}
This Post Has 0 Comments